Django Server
Introduction
In this part, we will present the Django server and how it works in detail. In our django app we have three apps and we use three services, the apps being the following:
- config, an app that configures the base user model for the app
- rest, an app that handles HTTP requests with DjangoRestFramework
- websocket, an app that handles websocket requests with the Daphne websocket server and we the services are:
- PostgreSQL the database to stores our users
- Redis the database that helps our app handle websocket requests
- Livekit an online service that eases the streaming of video
Server configuration
Django server by itself doesn’t have all the features to be a fully secure server, so we added CORS and CSRF to protect both the user and the backend from an attack.
PostgreSQL
PostgreSQL is one of the biggest and oldest projects to exist, with the purpose of being an ACID database, we use it to store our uses with a simple table:
Redis
Redis is fast and lightweight database that relies on the ram for its speed, we use it to handle large websocket connection when we will scale the django server to multiple replicas, allowing connections to be used by any replica instead of them being unique to a specific instance.
HTTP API
Authentication
We use a token based login system to authenticate our user, the JSONWebToken is a very effective way to authenticate and authorize users but with a single caveat: if the token is leaked, the user is compromised and the server can’t know it. JSONWebToken is a special string of characters, unique to the user and can only be generated by the django server, meaning any attempt of generating a token is useless. This token should be sent in each requests that requires a user to be logged in as it contains the userId and userType that allows the server to know who it is communicating with. And this very token is obtained by doing a GET request at the following endpoint:
The tokens also have a lifetime as a security measure, everyday the token expires and the user is required to authenticate again generating a new token.
Websocket API
We use Daphne to make the websocket server possible, it’s worth nothing that websocket is stateless and doesn’t have the features HTTP has like adding information to the body etc. There’s no builting authorization system only messages being sent in real-time over TCP, hence we made our own system.
Connection format and OpCodes
We use a system of Operator Codes called OpCode, and optionally send data with it, the format is as follows:
The system we made allows clear communication, and here’s a clear table of the available opcodes:
OpCode | Name | Sent by the server | Sent by the user |
---|---|---|---|
0 | Hello | yes | no |
1 | Heartbeat | no | yes |
2 | Heartbeat ACK | yes | yes |
3 | Identify | no | yes |
4 | Command | if the user is factory | if the user is operator |
5 | Status | if the user is not factory | if the user is factory |
6 | Event | yes | yes |
The sequence of these events is specific and is detailed in the diagram here:
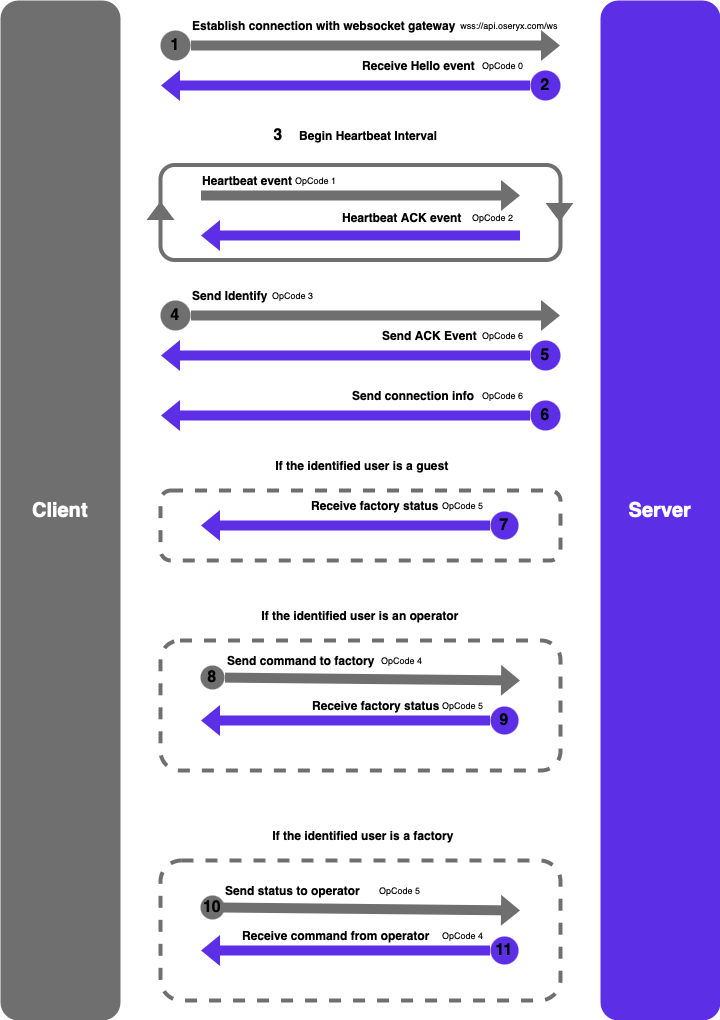
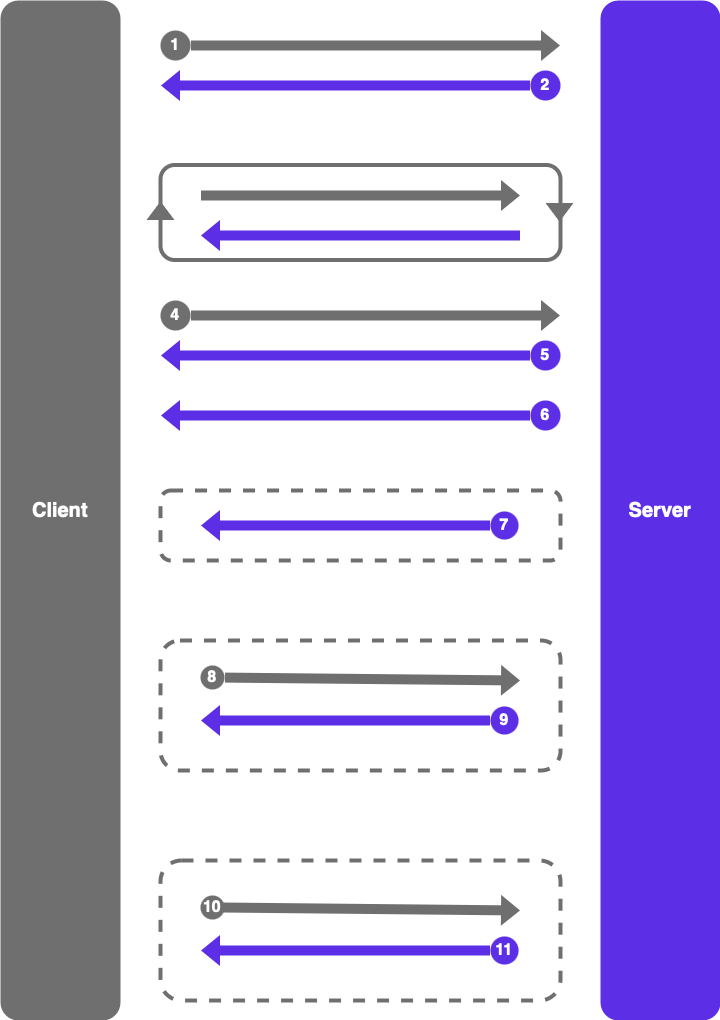
Relaying the requests
What our servers actually does is simply relaying the requests between the operators and the factories in a secure way, the factory can’t access the operator and vice versa, in fact the only entity known is the server itself. When a command is sent, the server firsts checks if the user is an operator, if so he broadcasts it to all the users of type factory, and the exact same process happens again when the factory sends the status.
Security measures
Out of security, if the connected user tries to make a single request where he doesn’t have the authorization to, the connection is immediately disrupted and he has to reconnect all over again. This prevents unwanted behavior and potentially finding a way to breach the server, the best measure is to not allow any altercation altogether.
Realtime Video Streaming
The final part is the ability to stream the camera in real-time, it’s good to see the status of the factory, but to see truly what happens is better, and for that we rely on WebRTC.
WebRTC
In our day to day life, two main protocols are used for streaming video and audio, the RTMP protocol used by Youtube, Twitch and other big streaming companies and WebRTC. WebRTC is the only real-time protocol, RTMP has a delay of approximately 5 seconds which makes it not suitable for our application, that is why WebRTC is used, it is a standard across big companies like Facebook, WhatsApp, Instagram, Discord etc. The adavantages are clear, but actually making the webrtc server is extremely difficult and with our time and constrains we preferred to use an external service that eases the work called Livekit.
Livekit
To actually stream we use the Livekit API, the user needs to make an HTTP request to the following endpoint:
The server will authorized the user if the token is sent with the request, and will generate a special Livekit API Token that allows users of type factories to send video in real-time, and users of any other type to only receive it.
Conclusion
Now that the django server is set, the core of our service is finished but the deployment isn’t simple, and security doesn’t stop in Django but is far more complex, that is what we will do in the next Chapter.